哈希空间
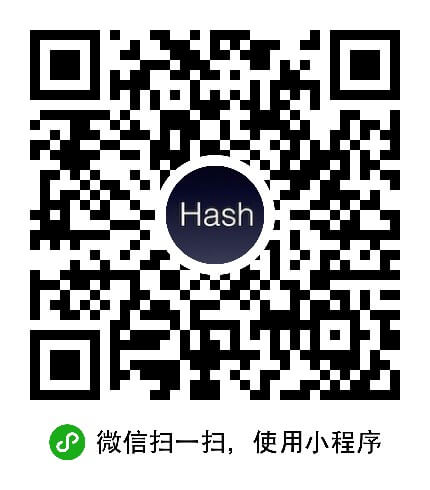
C语言WebSocket 代码例子
在 C 语言中连接 WebSocket 需要使用第三方库,例如 libwebsockets
。
以下是一个简单的示例代码,演示如何使用 libwebsockets
库连接 WebSocket:
#include <libwebsockets.h>
#include <string.h>
#include <stdio.h>
static int websocket_callback(struct lws *wsi, enum lws_callback_reasons reason, void *user, void *in, size_t len) {
switch (reason) {
case LWS_CALLBACK_CLIENT_ESTABLISHED:
printf("WebSocket client connected\n");
break;
case LWS_CALLBACK_CLIENT_RECEIVE:
printf("Received data: %s\n", (char *)in);
break;
case LWS_CALLBACK_CLIENT_WRITEABLE: {
char *message = "Hello, WebSocket server!";
unsigned char buf[LWS_SEND_BUFFER_PRE_PADDING + strlen(message) + LWS_SEND_BUFFER_POST_PADDING];
int len = sprintf((char *)&buf[LWS_SEND_BUFFER_PRE_PADDING], "%s", message);
lws_write(wsi, &buf[LWS_SEND_BUFFER_PRE_PADDING], len, LWS_WRITE_TEXT);
break;
}
case LWS_CALLBACK_CLOSED:
printf("WebSocket client closed\n");
break;
default:
break;
}
return 0;
}
int main() {
struct lws_context_creation_info info;
struct lws_client_connect_info connect_info;
struct lws_context *context;
struct lws *wsi;
const char *protocol = "ws";
const char *address = "localhost:8080";
int port = 80;
int use_ssl = 0;
int ret;
memset(&info, 0, sizeof(info));
info.port = CONTEXT_PORT_NO_LISTEN;
info.protocols = lws_protocols;
info.gid = -1;
info.uid = -1;
context = lws_create_context(&info);
if (!context) {
printf("Failed to create WebSockets context\n");
return -1;
}
memset(&connect_info, 0, sizeof(connect_info));
connect_info.context = context;
connect_info.address = address;
connect_info.port = port;
connect_info.path = "/";
connect_info.host = lws_canonical_hostname(context);
connect_info.origin = lws_canonical_hostname(context);
connect_info.protocol = protocol;
connect_info.ssl_connection = use_ssl;
wsi = lws_client_connect_via_info(&connect_info);
if (!wsi) {
printf("Failed to connect to WebSocket server\n");
return -1;
}
while (1) {
ret = lws_service(context, 10);
if (ret < 0) {
printf("WebSockets service failed\n");
break;
}
}
lws_context_destroy(context);
return 0;
}
这个示例代码使用了 libwebsockets
库的一些基本函数来实现 WebSocket 连接和数据收发。其中,websocket_callback
函数是 WebSocket 连接的回调函数,用于处理不同的事件。
请注意,这个示例代码仅作为演示用途,实际使用中可能需要根据具体情况进行修改。
本文 最佳观看地址:https://www.hashspace.cn/c-websocket-example.html 阅读 2260